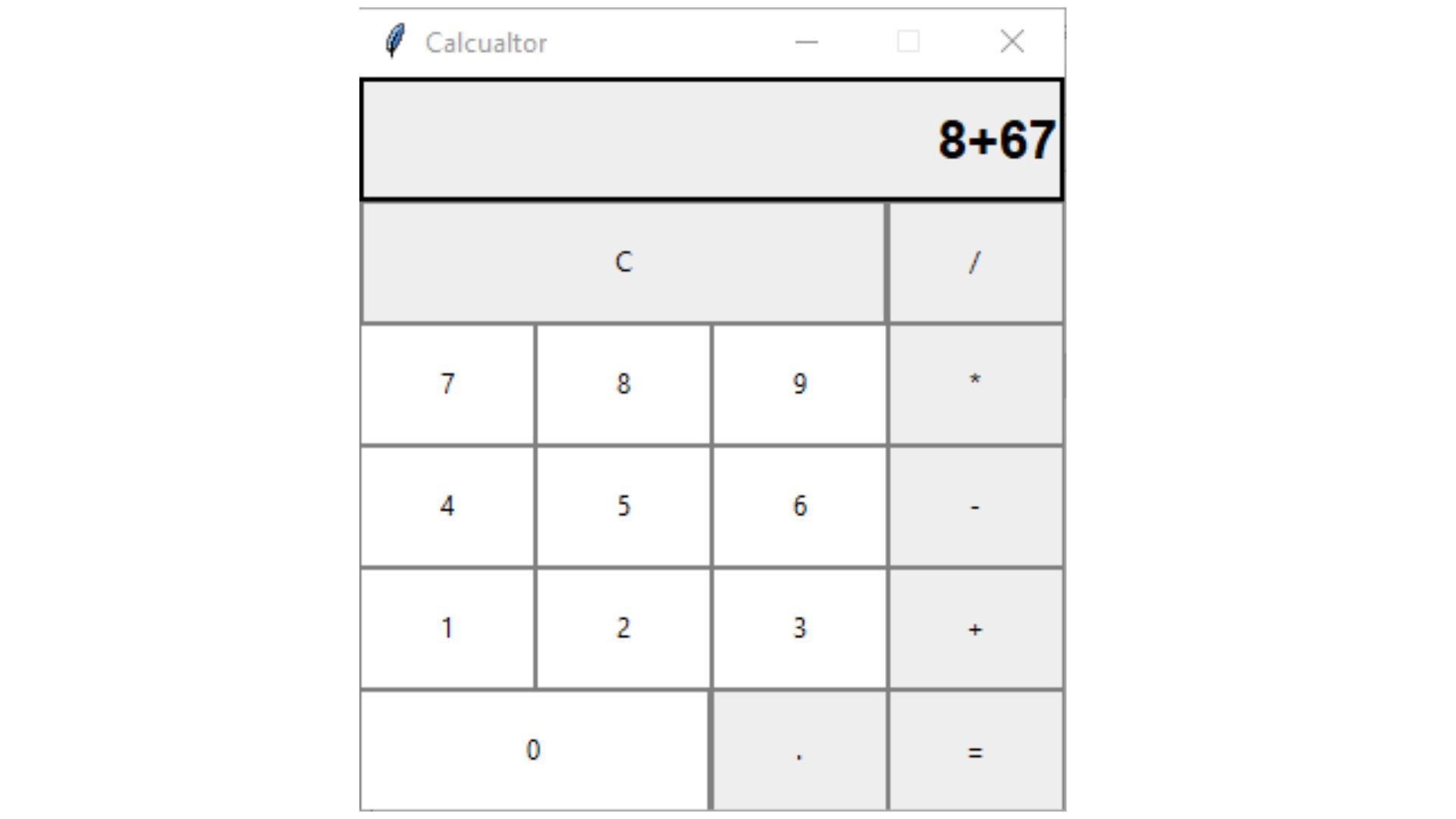
Why Build a Python Calculator?
Building a Python calculator has several benefits:
- Understand Python Basics: Operators, conditionals, loops, and functions are basic of Python and you will be using them in the program.
- Error Handling: You’ll find out how to handle incorrect user inputs and avoid program terminations.
- Code Reusability: Building a Python calculator helps you practice writing good-quality code that can be reused and is easily modularized.
- Confidence Boost: This project can be considered as demanding and only its completion should provide confidence when addressing more challenging tasks.
Setting Up Your Development Environment
The first course of action and therefore the initiation of this development process is to have the tools required for the project to be installed in your system.
- First, make sure that you have Python installed in your machine. Its installation can be performed from the official website of the Python programming language.
- For coding and debugging utilize an Integrated Development Environment (IDE), ranging from Visual Studio Code, PyCharm, or even the default IDLE.
Basic Calculator Functionality
Now let’s start with a basic calculator that performs four main operations: These basic operations involve addition, subtraction, multiplication, and division.
Step 1: Define the Operations
First define each arithmetic operation that the basic calculator can perform.
def add(a, b):
return a + b
def subtract(a, b):
return a - b
def multiply(a, b):
return a * b
def divide(a, b):
if b == 0:
return "Error: Division by zero is undefined."
return a / b
Step 2: Create a Menu System
In this project we can also implement a form or pop-up interface in order to enable the user to choose which option or operation of the system he or she wants to run.
def calculator():
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
choice = input("Enter choice (1/2/3/4): ")
if choice in ('1', '2', '3', '4'):
try:
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
except ValueError:
print("Invalid input! Please enter numerical values.")
return
if choice == '1':
print(f"The result is: {add(num1, num2)}")
elif choice == '2':
print(f"The result is: {subtract(num1, num2)}")
elif choice == '3':
print(f"The result is: {multiply(num1, num2)}")
elif choice == '4':
print(f"The result is: {divide(num1, num2)}")
else:
print("Invalid choice! Please select a valid operation.")
Step 3: Run the Calculator
In the end, to test the calculator, call the calculator
function:
if __name__ == "__main__":
calculator()
Save this code in a file named calculator.py
and run it. You now have a functional basic calculator!
Expanding to a Scientific Calculator
For the improved usability of the calculator, we can expand it for the following operations, exponentiation, square root, and trigonometric operations.
Adding New Functions
import math
def power(a, b):
return a ** b
def square_root(a):
return math.sqrt(a)
def sine(angle):
return math.sin(math.radians(angle))
def cosine(angle):
return math.cos(math.radians(angle))
def tangent(angle):
return math.tan(math.radians(angle))
Updating the Menu
Update the calculator
function to include these new operations.
def calculator():
print("Select operation:")
print("1. Add")
print("2. Subtract")
print("3. Multiply")
print("4. Divide")
print("5. Power")
print("6. Square Root")
print("7. Sine")
print("8. Cosine")
print("9. Tangent")
choice = input("Enter choice (1-9): ")
try:
if choice in ('1', '2', '3', '4', '5'):
num1 = float(input("Enter first number: "))
num2 = float(input("Enter second number: "))
if choice == '1':
print(f"The result is: {add(num1, num2)}")
elif choice == '2':
print(f"The result is: {subtract(num1, num2)}")
elif choice == '3':
print(f"The result is: {multiply(num1, num2)}")
elif choice == '4':
print(f"The result is: {divide(num1, num2)}")
elif choice == '5':
print(f"The result is: {power(num1, num2)}")
elif choice == '6':
num = float(input("Enter number: "))
print(f"The result is: {square_root(num)}")
elif choice in ('7', '8', '9'):
angle = float(input("Enter angle in degrees: "))
if choice == '7':
print(f"The result is: {sine(angle)}")
elif choice == '8':
print(f"The result is: {cosine(angle)}")
elif choice == '9':
print(f"The result is: {tangent(angle)}")
else:
print("Invalid choice! Please select a valid operation.")
except ValueError:
print("Invalid input! Please enter numerical values.")
Adding a User-Friendly Interface
To complement your calculator, you can add a graphical user interface which is GUI by using the Tkinter module.
GUI Implementation
import tkinter as tk
def click(event):
text = event.widget.cget("text")
if text == "=":
try:
result = eval(str(screen.get()))
screen.delete(0, tk.END)
screen.insert(tk.END, str(result))
except Exception as e:
screen.delete(0, tk.END)
screen.insert(tk.END, "Error")
elif text == "C":
screen.delete(0, tk.END)
else:
screen.insert(tk.END, text)
root = tk.Tk()
root.title("Calculator")
screen = tk.Entry(root, font=("Arial", 20), borderwidth=5, relief="solid")
screen.grid(row=0, column=0, columnspan=4)
buttons = [
"7", "8", "9", "+",
"4", "5", "6", "-",
"1", "2", "3", "*",
"C", "0", "=", "/"
]
row = 1
col = 0
for btn in buttons:
button = tk.Button(root, text=btn, font=("Arial", 18), relief="solid", padx=20, pady=20)
button.grid(row=row, column=col, padx=5, pady=5)
button.bind("<Button-1>", click)
col += 1
if col > 3:
col = 0
row += 1
root.mainloop()
This code gives us a small example of a GUI-based calculator. If you don’t want to open it fully, you can run it and see a window with several buttons for basic operations.
Testing and Debugging
- Boundary Cases: Perform this test with possibilities on the extreme condition that is, /0 or 0/, and testing large numbers.
- Invalid Inputs: Unleash your calculator’s error-resistance with respect to input values. Try checking the output by giving invalid input for normal arithmetic operations.
- Functionality Checks: Check all functional operations to be on the safer side - including both basic and scientific calculations.