C++ Fundamentals: Understanding the Basics
Difference Between C and C++ Programming Languages
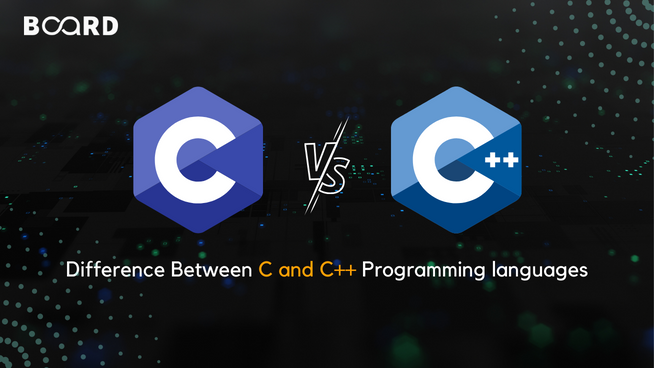
The Programming languages C and C++ are used to create a variety of things, including operating systems, databases, games, and apps. Although C and C++ seems to have similar elements, they differ in terms of functionality and application. Because it is a structured programming language, C somehow doesn't provide objects and classes. The object-oriented programming language C++ is an improved version of the C programming language. We'll talk about the differences between C and C++ programming languages in this blog. We'll also comprehend the distinctions between C and C++.
C Programming Language
The C programming language was created by Dennis Ritchie in 1972 at Bell Laboratories. Several object-oriented scripting languages, including C++, a superset of C, and Java, an extension of C++, have their roots in the C language. The language was initially created to let people can write and create operating system-building programmes. All of the programmes and utilities that make up UNIX OS were initially built in C. Additionally, the C programming language was used to create programmes like compilers, text editors, assemblers, drivers, databases, and other useful utilities.
What is C Programming Language?
The C programming language is also sometimes referred to as God's programming language. One reason for this is that it serves as the foundation for many other programming languages. A user can conceivably learn some other programming language if they are familiar with the C language. It is different from other programming languages in that it contains a few extra features.
The "Procedural Programming" concept forms the basis for the C language. This indicates that this programming language offers procedures a higher priority over data.
Several issues occurred as a result of the procedural programming principle's emphasis on procedures rather than data, particularly with regards to expanding and sustaining software. Its replacement, C++, came into play at that point. This provides its data and related functions in it top priority. The C programming language is also incompatible with other general-purpose programming languages. There are chances that you will face interview questions related to C programming in any tech domain.
Features of C Programming
1. Simple and Efficient
2. Fast
3. Portability
4. Extensibility
5. Function-rich libraries
6. Dynamic Memory Management
7. Modularity With Structured Language
8. Mid-Level Programming Language
9. Pointers
10. Recursion
Applications of C programming
1. Embedded System
2. Google
3. Design of a Compiler
4. Operating Systems
5. GUI (Graphical User Interface)
6. New Programming Platforms
7. MySQL
8. Gaming and Animations
9. Mozilla Firefox and thunderbird
10. Translators of high-level languages into machine language
C Programming Language Example
We Will discuss some of the C programming languages:
1. Hello World Program
#include <stdio.h>
int main(){
printf("Hello World!!");
return 0;
}
Output:
Hello World!
2. Program check Leap Year
#include <stdio.h>
int main() {
int year;
printf("Enter a year: ");
scanf("%d", &year);
// leap year if perfectly divisible by 400
if (year % 400 == 0) {
printf("%d is a leap year.", year);
}
// not a leap year if divisible by 100
// but not divisible by 400
else if (year % 100 == 0) {
printf("%d is not a leap year.", year);
}
// leap year if not divisible by 100
// but divisible by 4
else if (year % 4 == 0) {
printf("%d is a leap year.", year);
}
// all other years are not leap years
else {
printf("%d is not a leap year.", year);
}
return 0;
}
Output:
Enter a year: 2016
2016 is a leap year.
3. Program to reverse a number
#include <stdio.h>
int main() {
int n, reverse = 0, remainder;
printf("Enter an integer: ");
scanf("%d", &n);
while (n != 0) {
remainder = n % 10;
reverse = reverse * 10 + remainder;
n /= 10;
}
printf("Reversed number = %d", reverse);
return 0;
}
Output:
Enter an integer: 1234
Reversed number: 4321
4. Program to check whether a number is prime or not
#include <stdio.h>
int main() {
int n, i, flag = 0;
printf("Enter a positive integer: ");
scanf("%d", &n);
// 0 and 1 are not prime numbers
// change flag to 1 for non-prime number
if (n == 0 || n == 1)
flag = 1;
for (i = 2; i <= n / 2; ++i) {
// if n is divisible by i, then n is not prime
// change flag to 1 for non-prime number
if (n % i == 0) {
flag = 1;
break;
}
}
// flag is 0 for prime numbers
if (flag == 0)
printf("%d is a prime number.", n);
else
printf("%d is not a prime number.", n);
return 0;
}
Output:
Enter a positive integer: 31
31 is a prime number
C++ Programming Language
The object-oriented C++ programming language was created as a C language extension. As a result, in addition to the procedural language capabilities from C, C++ also supports object-oriented features. The Object-Oriented Programming paradigm serves as the foundation for the C++ programming language (OOPs). The characteristics of object-based programming are under the umbrella of object-oriented programming.
What is C++ Programming Language?
C++ programming language combines all the attributes of object-based programming and overcomes its constraints through the use of inheritance, allowing you to use programming to solve problems based on real-world scenarios. Object-oriented programming was developed to address the shortcomings of conventional programming methods. The OOPs approach was created based on a few ideas that help it accomplish its goal of outperforming the shortcomings or shortcomings of conventional programming methodologies.
Examples include abstraction, encapsulation, polymorphism, inheritance, and more.
The C++ language and the C language are very similar. This language is so compatible with C that it can execute 99 percent of C programmes without the need to modify their source codes. An object-oriented programming language is C++. In comparison to the C language, it is safer and better organized. It is easy to learn and thus help developers to get started with work on some innovative project ideas.
Features of C++
1. OOP(Object Oriented Programming)
2. Simple
3. Platform Dependent
4. Mid-Level Programming Language
5. Speed
6. Compiler-based
7. Dynamic Memory Allocation
8. Existence of Libraries
9. Case Sensitive
10. Multi-threading
Applications of C++ Programming
1. GUI based Applications
2. Games
3. Operating System
4. Web Browsers
5. Libraries
6. Banking Applications
7. Cloud/Distributed System
8. Databases
9. Embedded Systems
10. Compilers
11. Switches
C++ Language Example
We Will discuss some of the C++ programming languages:
1. Hello World Program
#include <iostream>
int main() {
std::cout << "Hello World!";
return 0;
}
Output:
Hello World!
2. Program to check Leap Year
#include <iostream>
using namespace std;
int main() {
int year;
cout << "Enter a year: ";
cin >> year;
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
cout << year << " is a leap year.";
}
else {
cout << year << " is not a leap year.";
}
return 0;
}
Output:
Enter a year: 1900
1900 is not a leap year.
3. Program to reverse a number:
#include <iostream>
using namespace std;
int main() {
int n, reversed_number = 0, remainder;
cout << "Enter an integer: ";
cin >> n;
while(n != 0) {
remainder = n % 10;
reversed_number = reversed_number * 10 + remainder;
n /= 10;
}
cout << "Reversed Number = " << reversed_number;
return 0;
}
Output:
Enter an integer: 1234
Reversed number: 4321
4. Program to check whether a number is prime or not
#include <iostream>
using namespace std;
int main() {
int i, n;
bool is_prime = true;
cout << "Enter a positive integer: ";
cin >> n;
// 0 and 1 are not prime numbers
if (n == 0 || n == 1) {
is_prime = false;
}
// loop to check if n is prime
for (i = 2; i <= n/2; ++i) {
if (n % i == 0){
is_prime = false;
break;
}
}
if (is_prime)
cout << n << " is a prime number";
elsecout << n << " is not a prime number";
return 0;
}
Output:
Enter a positive integer: 31
31 is a prime number
To get a detailed knowledge about C++ you can opt for Data Structures and Algorithms with C++ Course and get a certifications to boost your career.
Difference between C and C++
Similarities Between C and C++
- The syntax of the two languages is identical.
- Both languages share the same code structure.
- Both languages are constructed similarly.
- They use the same fundamental syntax. The majority of the operators and keywords found in C are also found in C++ and perform the same functions.
- Although the underlying grammar of C++ and C are the same, C++ has a significantly longer syntax.
- Both have a basic memory model that closely resembles the hardware.
- Both languages share the same concepts of a stack, heap, file-scope, and static variables.
With the knowledge of C and C++, you can get a basic understanding of programming and can get best career options.
C vs C++: Which to Pick?
There are some circumstances where C or C++ might be the preferable choice.
At the professional level, C++ is the language that is used the most frequently worldwide. As was already stated, C++ proficiency is a need for many programming jobs in the workforce.
It's uncommon to find a program written entirely in C, and more apps are written completely in C++. However operating systems using C as their primary language are recognized to incorporate C++ in other places to increase flexibility and depth.
If you're a complete rookie to programming, mastering object-oriented C++ will provide your abilities that are applicable to other programming languages, OOPs with Java, JavaScript, and Python being the best examples.
Actually, C++ is a superset of C. In a.cpp file, you can write C code. C++ can make use of libraries that C can. You must utilise C++ if you want to design applications that use oops principles, generic programming, and sophisticated data structures.
Additionally, because C++ includes the majority of the necessary data structures as libraries, it is superior for writing code quickly and in less lines. C++ is still an option if you're dealing with kernel-level programming, but since C is a simpler language and has a lighter compiler than C++, it will likely be utilised more frequently.
Conclusion
Simply said, the major distinction between C and C++ is that the former is a procedural language without support for objects or classes, while the other is a blend of procedural and object-oriented programming languages. You can choose which language is better for your projects by understanding the features, applications, drawbacks, and differences between C and C++.