C Programming: From Basic Syntax to Advanced Concepts
Function Pointers in C
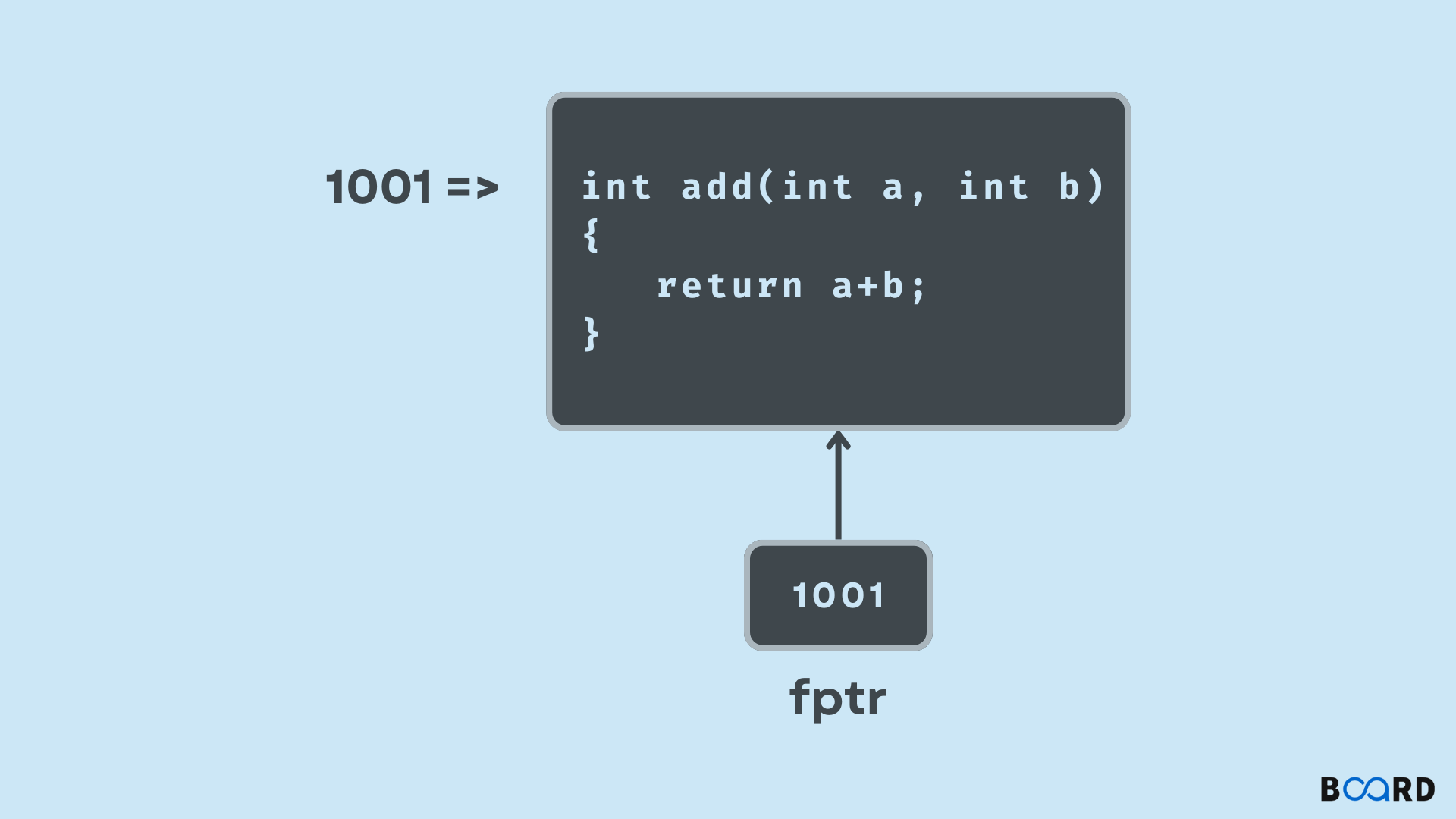
C is probably the most popular programming language, which is universal and allows precise control over functional resources. One of those extra features called function pointers extends its capabilities even further. Function pointers as you can see often may seem a little complicated, especially to a new programmer but makes your programming arsenal a lot stronger if mastered.
This blog post is a complete resource for learning about function pointers in C language, along with various uses examples and real use cases.
Introduction to Function Pointers
Just like in C, these are the variables that contain the address of another variable in the computer memory. Function pointers are of pointers that point to the address of a function they need to point to. Consider pointers to variables, these act as references to the data type you want to work with, and in the same way function pointers in C allow you to call functions.
Function pointers in C can be used to pick functions at runtime, for callbacks and event handling, as an alternative to numerous case expressions or if-else, in plugin design and finite state systems to name a few.
Declaring Function Pointers in C
Just to declare a pointer to a function could be a little mixed up at first sight. The c function pointer syntax is:
return_type (*pointer_name)(parameter_list);
For example, to declare a pointer to a function that takes two integers as parameters and returns an integer:
int (*operation)(int, int);
Here, int is the data type of the function and it is also the data type of the variable operation which has been declared as pointer to function. The last part (int, int) indicates that the functions takes two int arguments.
Assigning Functions to Pointers
A function pointer variable can hold address of any function that has the type as that of the pointer. For example:
int add(int a, int b) {
return a + b;
}
int main() {
int (*operation)(int, int); // Declare function pointer
operation = add; // Assign address of add() to operation
printf("Sum: %d\n", operation(5, 3)); // Call add() through pointer
return 0;
}
In the following example, the function pointer operation is pointed to the address of add functions and used to call the functions.
Calling Functions Through Pointers
When using pointer to a function we can call this function directly using the pointer followed by the arguments in the parenthesis. For example:
result = (*pointer_name)(arguments);
The parentheses around *pointer_name are optional in most cases, so you can also write:
result = pointer_name(arguments);
Thus, both forms are used in default and switch between the two.
Practical Applications of Function Pointers in C
One of the most popular was was dynamic function selection. For example:
#include <stdio.h>
int add(int a, int b) { return a + b; }
int subtract(int a, int b) { return a - b; }
int main() {
int (*operation)(int, int);
char choice;
printf("Enter operation (+ or -): ");
scanf(" %c", &choice);
operation = (choice == '+') ? add : subtract;
printf("Result: %d\n", operation(10, 5));
return 0;
}
Function pointers in C are also required in order to enable callbacks. For example:
#include <stdio.h>
void greet(void (*callback)(void)) {
printf("Hello! ");
callback();
}
void sayGoodbye() {
printf("Goodbye!\n");
}
int main() {
greet(sayGoodbye);
return 0;
}
One of the real uses is the arrays of function pointers in C that leads to simpler designs. For example:
#include <stdio.h>
int add(int a, int b) { return a + b; }
int subtract(int a, int b) { return a - b; }
int multiply(int a, int b) { return a * b; }
int main() {
int (*operations[3])(int, int) = {add, subtract, multiply};
printf("Addition: %d\n", operations[0](10, 5));
printf("Subtraction: %d\n", operations[1](10, 5));
printf("Multiplication: %d\n", operations[2](10, 5));
return 0;
}
They are also used in plugin systems to load and execute any module at runtime dynamically.
Advantages and Disadvantages
Some of the advantages of using function pointers in C include dynamic function selection, less code repetition, as well as a number of system enhancing features such as call back mechanisms and event handling. However, they may complicate the code due to their syntax and usage, might contain an error if used in the wrong context and finally, when it comes to debugging, plunges a programmer into a lot of difficulties.
Conclusion
For reaching in flexibility and application, function pointers in C are a unique feature which can greatly enhance the design of your programs. While first it might seem puzzling, once you grasp the concept, you’ll discover numerous opportunities in your programming arsenal. With examples use them often and try out how they can be applied in order to fully understand the regulations. Happy coding!
FAQs: Function Pointers in C
How do you declare a function pointer in C?
The C function pointer syntax is as follows:return_type (*pointer_name)(parameter_list);
What are the common uses of function pointers in C?
Function pointers can be implemented in callback functions, for dynamic function dispatch, for decision making structures, and for creating function pointer arrays.
Can a function pointer in C point to any function?
A function pointer points to any function which has been defined with such same data types.
Are function pointers in C safe to use?
Function pointers are powerful yet if not used correctly, they cause undefined behavior. Due to the simple syntax, there may be no error if the correct match of the type and initialization are carried out.