FuzzyWuzzy Python Library
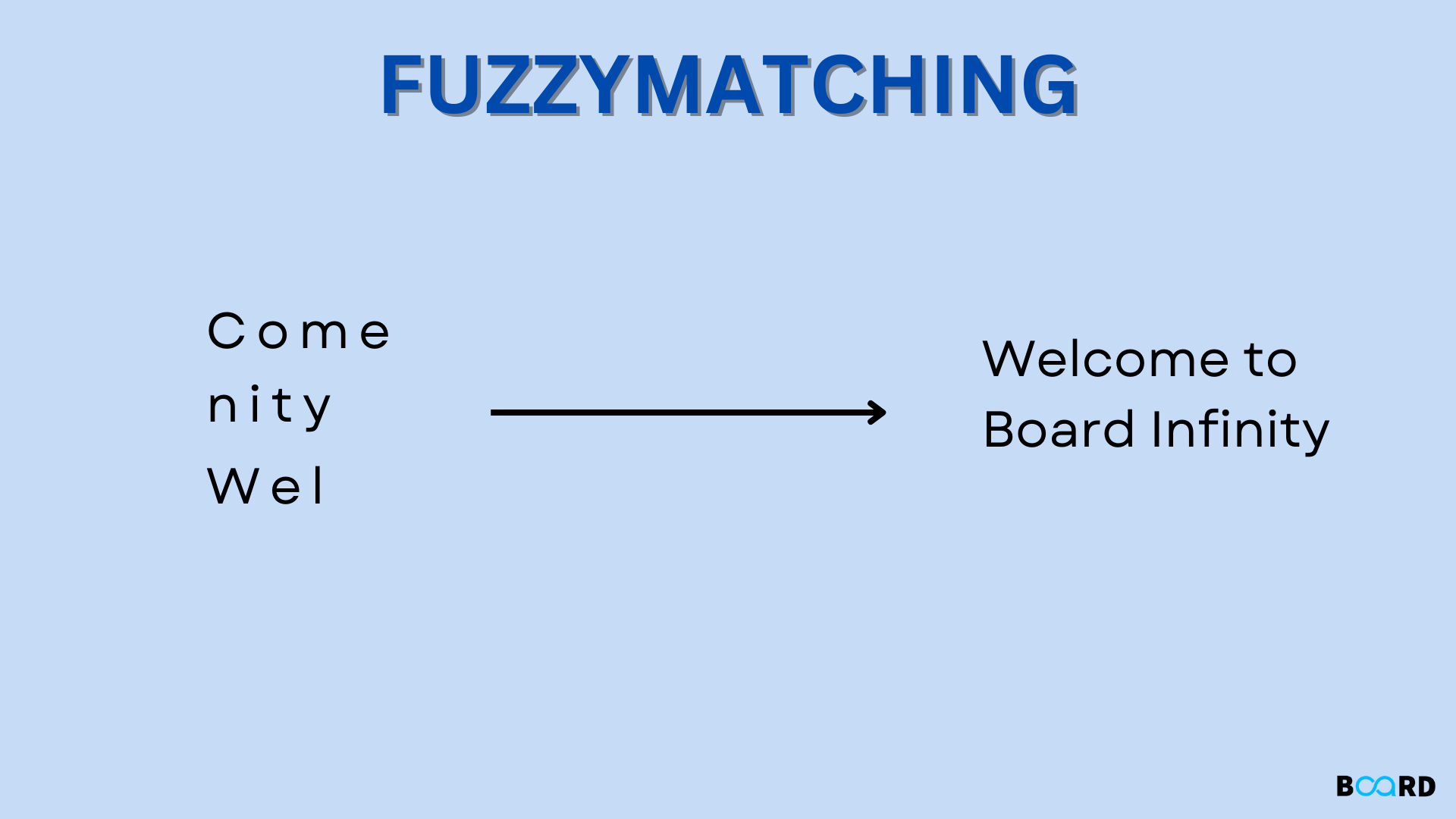
Introduction
Python's FuzzyWuzzy library is used for string matching. Finding strings that match a specified pattern is known as fuzzy string matching. In essence, Levenshtein Distance is used to determine the differences between sequences.
By using SeatGeek's service to find sports and concert tickets, FuzzyWuzzy has been created and made available to the public. Their blog post's discussion of their initial use case.
FuzzyWuzzy is one of the best library used for string matching, where we can have a score out of 100 indicating the similarity index.
Installation Syntax
FuzzyWuzzy library can be installed using the following syntax:
Imports of FuzzyWuzzy library can be done using the following syntax:
Parameters
Parameters used by Python filter() function is enlisted below:
- function: it is the function that tests if each element of a sequence true or not.
- sequence: it is the sequence which needs to be filtered, it can be sets, lists, tuples, or containers of any iterators.
Return Value
This function returns an filtered iterator that can be iterated upon.
Simple Ratio Module
Ratio module can be used for comparing the String values and getting a score of how the strings are similar.
Partial Ratio Module
Partial Ratio can be used for comparing the String values partially and getting a score of how the strings are similar.
Token Sort Ratio
Token Sort ratio sorts and compare the string values
WRatio
WRatio is used to compare string values by handling uppercase and lowercase letters.
Example: Lets take an full code example where we are comparing strings using this library.
Output: