Understanding Algorithm: Complexity and Performance
How to Build a TreeMap?
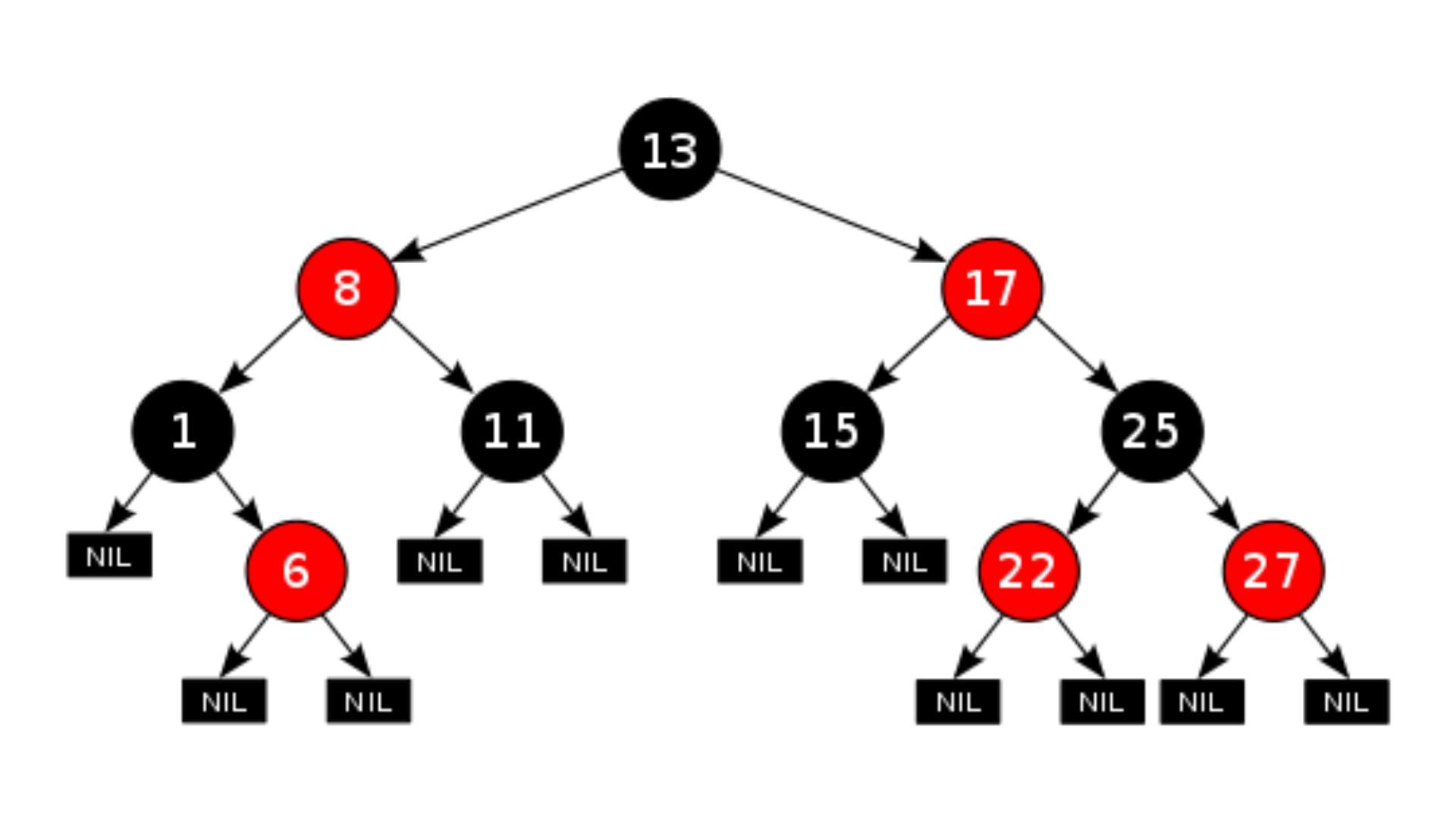
What is TreeMap?
In Java, TreeMap implements Map, NavigableMap, and AbstractMap classes. Depending on the constructor, the map gets sorted based on its natural ordering or based on a Comparator. Using a TreeMap, you can efficiently store key/value pairs sorted and provide rapid retrieval.
In contrast to a hash map, a tree map guarantees that its elements will get sorted in ascending key order.
Creating a TreeMap requires importing the java.util.TreeMap package. After importing the package, here is how to create a TreeMap in Java.
TreeMap<Key, Value> numbers = new TreeMap<>();
The code above creates a TreeMap named numbers without any parameters. In this case, TreeMap items get sorted ascendingly.
The Treemap Constructor
#1. public TreeMap()
This function constructs a new, empty tree map based on its keys' natural ordering. All keys inserted into the map must implement the Comparable interface. A key must implement a Comparable interface when inserted into the map.In addition, each of these keys must be mutually comparable: k1.compareTo(k2) must not throw a ClassCastException for any keys k1 and k2 in the map.
When a key gets inserted into the map that violates this constraint (for example, putting a string key into a map with integer keys), the put(Object key, Object value) method throws a ClassCastException.
#2. public TreeMap(Comparator<? super K> comparator)
This comparator helps construct an empty tree map. There must be a mutual comparison between all keys inserted into the map: comparator.compare(k1, k2) must not throw a ClassCastException for any keys k1 and k2 in the map.
As soon as the user attempts to insert a key that violates this constraint, the put(Object key, Object value) method throws a ClassCastException.
Parameters:
- comparator -
the comparator will order this map. It will use the natural ordering of the keys if null.
#3. public TreeMap(Map<? extends K,? extends V> m)
This function creates a new tree map with the same mappings as the given map, ordered based on its natural order. The new map must implement a Comparable interface on all keys inserted into the new map. In addition, the keys in the map must be mutually comparable: k1.compareTo(k2) must not throw a ClassCastException for any keys k1 and k2 in the map. This method runs in n*log(n) time.
Parameters:
- m - This map is to be used to place mappings
Throws:
- ClassCastException -
If m has keys that aren't comparable, or if they aren't mutually comparable
- NullPointerException -
A null map is specified
#4. public TreeMap(SortedMap<K,? extends V> m)
This function creates a new tree map with the same sorting and mappings as the sorted map supplied. It takes linear time to run this method.
Parameters:
- m -
This map contains mappings that should get placed in this sorted map and a comparator that gets used for sorting.
Throws:
- NullPointerException -
In the case of a null map