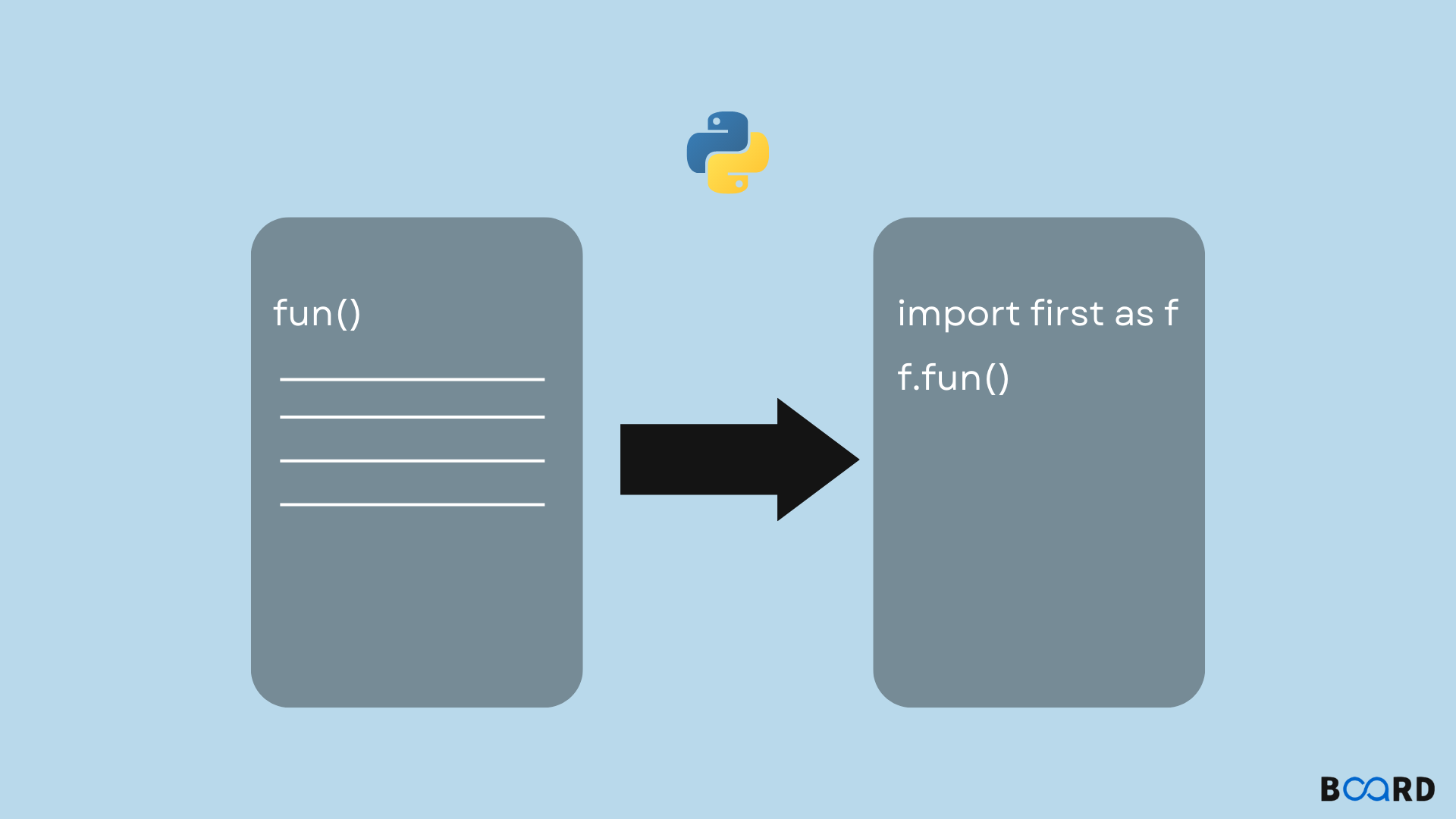
Organizing your work in more files (modules) is crucial for bigger Python projects to provide better output and be easier to understand. In Python, you can call a class that you have defined in a different file into your current file using Python’s import system. Here is a process analysis on how to achieve this in detail.
Example 1:
This section will demystify how to import a class using the import keyword.
To use the class in another file, we can use the import keyword to call the entire module at once. After importing the module, it is possible to use the class through the module name.
Suppose you have a file person.py containing the following class:
# person.py
class Person:
def __init__(self, name):
self.name = name
def greet(self):
print(f"Hello, my name is {self.name}.")
To use the Person class in another file (e.g., main.py), you would import the whole module like this:
# main.py
import person # Import the entire person module
# Use the Person class from the Person module
p = person.Person("Alice")
p.greet()
Output:
Hello, my name is Alice.
As in the previous example, the whole person module is imported; the Person class is accessed by referencing the module name and class name separated by a dot.
Example 2:
In general, importing defines a name that points to an object that was previously created Using from module import class
# main.py
# Import the Person class directly
from person import Person
# Now you can use the Person class without referencing the module name
p = Person("Bob")
p.greet()
Output:
Hello, my name is Bob.
In the case where you are only interested in a particular class from the module the from …import … syntax can be used to import just the class so that the code is cleaner and you do not always have to refer to the name of the module.
Here we have directly imported Person from person.py, so the concept of the person module has been done away with.
What is the Difference Between import module and from module import class
Import module: This method imports the whole module. When it comes to using any class or function from that module you have to write the name of the module again and again. For example ‘the name of the module dot the name of the class’. This comes in handy when you require an instance of a couple of classes or multiple functions from the same module, without polluting the namespace.
When to use each approach: There are three cases when using an import module for Python code:
- When you need to import several items in a module;
- When you wish to support code readability, and
- Want to keep the module’s namespace
When you need to import only a specific class or function Namespace from the module and for repeated class writing, instead of typing, use from module import class in Python.
Import Class Python: With an Alias
from module_name import class_name as alias
Example:
from person import Person as P
# Alias 'Person' as 'P'
p = P("Charlie")
p.greet()
# Output:
Hello, my name is Charlie.
How the file structure affects imports
The way you structure your project’s files affects how you import class in Python. Here's a breakdown:
Absolute Imports:
These are imports where you specify the full path from the root of your project. They are simple and clear.
Example project structure:
my_project/
├── main.py
└── models/
└── person.py
To import Person from person.py inside the models folder, you would use an absolute import:
from models.person import Person
Absolute imports are preferred because they are explicit and help avoid ambiguity.
Relative Imports:
These imports are used when files are within the same package or directory. They specify the location of the module relative to the current script.
Example:
my_project/
├── main.py
└── models/
└── __init__.py
└── person.py
Inside the models/__init__.py file, you could use a relative import to bring in the Person class from person.py:
from .person import Person
Relative import from the same directory
Relative imports are useful when working within packages and submodules but should be avoided for top-level scripts, as they can be less clear.
Common Issues When Importing Classes in Python
Python does have a great import system but it has a couple of bugs mostly appearing in more complex projects where many modules rely on one another. Two of the most recognized issues that developers face are circular imports, and ImportError or ModuleNotFoundError. We can examine each of these in more detail and some possible solutions to each.
Circular Imports
A circular import is a situation whereby two or more modules attempt to import each other either directly or through other modules cyclical in nature. For instance, Module A has imported Module B, while Module B, in the same way, imports Module A. This makes it to an extent where Python is at a deadlock trying to load the dependencies as it is stuck in a cycle between the two modules.
The Problems That Circular Imports Bring
- Import Timing Issues: In Python, when Python imports a module, Python loads the described module and then also runs all the code contained in the module. There is a problem in Python when a module is importing something from another module, which in turn imports the original module, Python will attempt to load the first module again before the first one is loaded fully. All this results in an incomplete or a broken import.
- Partial Imports: Sometimes the whole module is imported half-heartedly or in some other cases Python refuses to import a certain module at all and then when an attempt is made to use one of the classes or function from the particular module, an AttributeError or an ImportError may result.
How to deal with circular import problems
To resolve or avoid circular imports, here are a few strategies:'
1. Rearrange the Imports:
Much of the time, it is just possible to refactor the code and eliminate the circular dependencies, and it will cure the issue. For instance, you can experiment with repositioning the import statement within the function or the method; importing a component is indeed the signal of its actual use. By delaying the import, Python doesn't attempt to load the module until it’s necessary, reducing the chance of a circular import.
# In module_a.py
def some_function():
from module_b import ClassB # Import inside the function to delay it
obj_b = ClassB()
2. Use Python’s importlib for Dynamic Imports:
In case circular import cannot be avoided, there is a form of import that is dynamic and might be done at runtime as opposed to at the start of a script as is usually done, this is through using the importlib toolbox. This can help to resolve the vicious circles It helps to break cyclical dependencies as in the following examples: In this way, the import of the module_b takes place while they are imported dynamically and it does not raise the problem of circular import.
# In module_a.py
import importlib
def some_function():
module_b = importlib.import_module('module_b')
obj_b = module_b.ClassB()
How to debug and resolve these errors
1. Check for Typos:
That is the reason why the most frequent source of import errors is a typo in a module or class name. Ensure you are typing everything correctly including capitalization because Python is case sensitive.
from math import Sqrt # Typo: 'Sqrt' should be 'sqrt’
2. Check the Module Path:
If you’re confronting a ModuleNotFoundError, the first thing to do is to look at whether that given module is installed and imported into the project. As such, you can verify Python’s search path (sys.path) to make sure the directory with the module is on it.
import sys
print(sys.path)
# This shows the directories Python searches for modules
3. Ensure the Module is Installed:
If you are getting ModuleNotFoundError while attempting to import a third-party module, just check that it is installed. You can install it using pip.
pip install module_name
4. Verify Dependencies:
They involve checking any third-party libraries and making sure all the necessary dependencies are installed appropriately and to date. In order to find out all of the installed packages and their versions, type pip freeze.
pip freeze