JSON file in Python: Read and Write
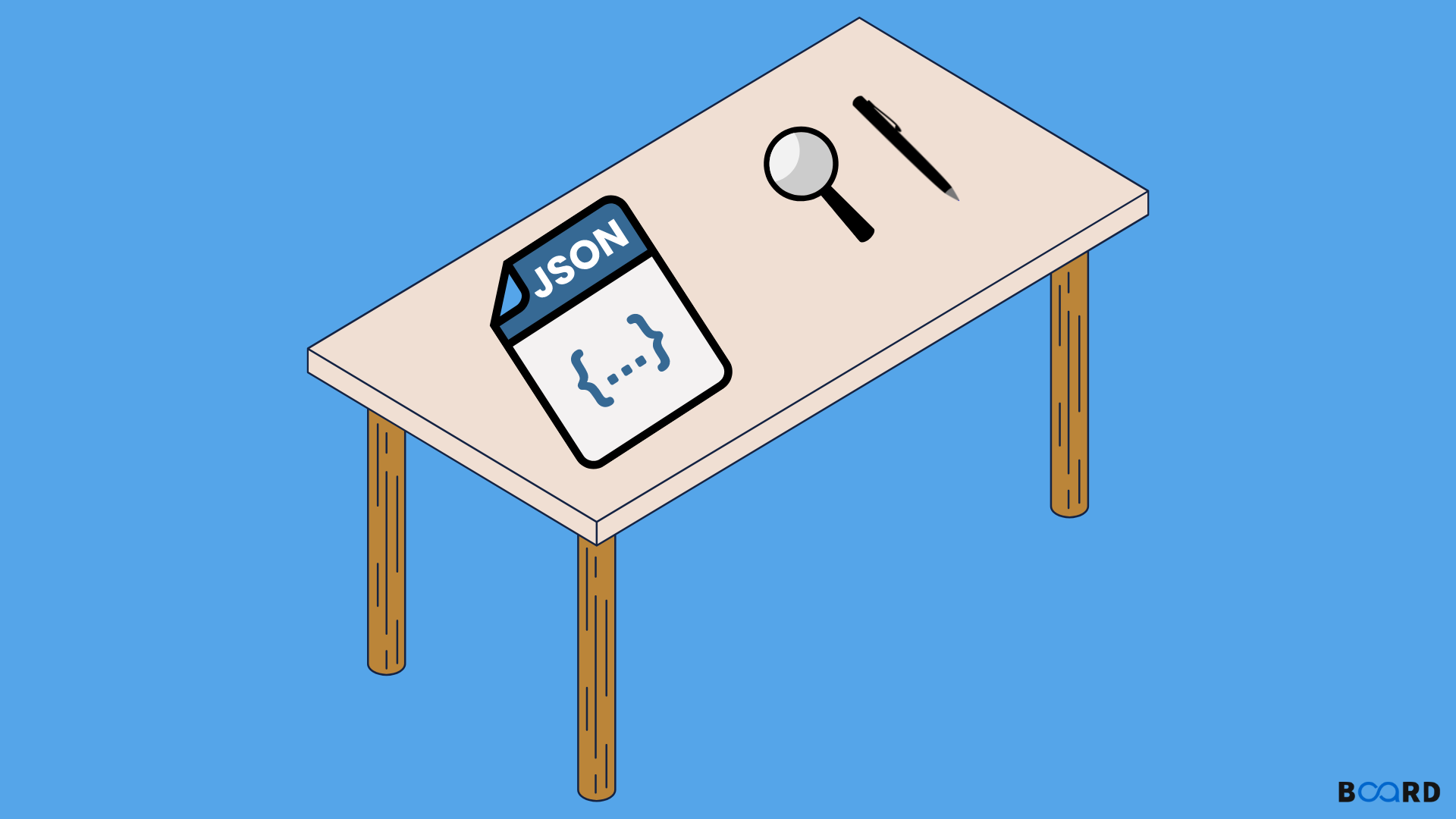
What is JSON?
JSON stands for JavaScript Object Notation, it is a lighter thin language used for the encoding for the data interchange system that is easy for the humans as well as providing a simple structure for the machines. It is derived from JavaScript’s object notation syntax and is structured data that consists of key-value pairs.
Basic Structure of JSON
- Objects: These are enclosed in curly braces {} and contain key-value pairs.
- Arrays: An array is an organized list of values placed in a bracket [] also known as a list.
- Data Types: JSON uses objects and arrays similarly to other programming languages; the value can be a string; number; Boolean (true/false); array; object; or null.
Example of a JSON object:
#python write json object to file
{
"name": "John",
"age": 30,
"isStudent": false,
"courses": [
"Math",
"Science"
]
}
- "name", "age", "isStudent", and "courses" are the keys.
- "John", 30, false, and ["Math", "Science"] are the values.
Working with JSON in Python
Python has a built-in JSON module that provides two main functions for working with JSON data: json.load() for reading purposes and json.dump() for writing purposes. Now let's look at effective ways of employing these functions.
Reading JSON from a File
To read from a file in Python using JSON the easiest method is using the json.load() method. This function parses the content of a JSON file and transforms it into a Python dictionary (or a list, according to JSON configuration).
Example:
import json
# Open and read the JSON file
with open('data.json', 'r') as file:
data = json.load(file)
# Print the content of the JSON file
print(json.dumps(data, indent=4))
- The code json.load(file) retrieves the contents of the file and shows its result in the form of a Python dictionary.
- If the JSON file is an array the method will return a Python list.
Error Handling
We believe that working with files always involves error handling. Issues can be something as simple as the file not being found, or something as complex as the content of the JSON file being read as invalid.
import json
try:
with open('data.json', 'r') as file:
data = json.load(file)
print(data)
except FileNotFoundError:
print("The file was not found.")
except json.JSONDecodeError:
print("The file contains invalid JSON.")
- If the file doesn’t exist then FileNotFoundError is raised.
- json.JSONDecodeError happens when the file is not a valid JSON file with respect to syntax.
Writing JSON to a File
When you have either modified or created Python data including a dictionary or list, you might wish to write this data back to a JSON file. The json.dump() function is another function in JSON that enables the writer to serialize and write Python JSON objects to a file.
Example:
#write json file python
import json
try:
# Open and read the JSON file
with open('data.json', 'r') as file:
data = json.load(file)
print(json.dumps(data, indent=4)) # Beautify the printed JSON
except FileNotFoundError:
print("The file was not found.")
except json.JSONDecodeError:
print("The file contains invalid JSON.")
- Json.dump writes a JSON object to a file by using the parameters data and files to reference each object.
- indent parameter enhances the format of the JSON output to have those indentations and spaces to make it more human-friendly.
Formatting Options:
Indentation: Estimate the indent of the JSON string which will make the JSON string more easily understandable and readable. Indents = 4 nests the data structures making them have additional spacing.
- Sorting Keys: You can also use the sort_keys=True parameter for the keys to be sorted in alphabetical order.
#format and write json file in python
json.dump(data, file, indent=4, sort_keys=True)
This will make sure that the keys are ordered alphabetically during the formation of the output JSON file.
Pretty Printing JSON
Often when dealing with large amounts of JSON data you might wish to add white space in order to make diagnostics or logs more readable. The json.dumps() function is used for pretty formatting of JSON data instead of writing it in a file.
Example:
#pretty write json file in python
import json
# Sample Python dictionary
data = {
"name": "John",
"age": 30,
"isStudent": False,
"courses": ["Math", "Science"]
}
# Convert Python dictionary to JSON string with pretty formatting
json_string = json.dumps(data, indent=4)
# Print the formatted JSON string
print(json_string)
- json.dumps() is the JSON data serialization method in Python that converts a Python object to a JSON Object.
- It is important that it adheres to a formal layout and this is enabled by setting the indent parameter.
Reading and Writing JSON with Strings (Using json.loads() and json.dumps())
Most of the time you will work with JSON data in files, but sometimes you may need to work with JSON strings (for example, when working with API data).
- json.loads(): Transforms JSON string into Python object, which can be often a dictionary.
- json.dumps(): Persists a Python object to form a JSON string.
Example:
#read and write json file in python
import json
# JSON string
json_string = '{"name": "Jane", "age": 28, "isStudent": true}'
# write json file in python to Python dictionary
data = json.loads(json_string)
print("Converted to Python dictionary:")
print(data)
# Convert Python dictionary back to JSON string with pretty formatting
json_output = json.dumps(data, indent=4)
print("\nConverted back to formatted JSON string:")
print(json_output)
- json.loads() converts the JSON given string into a Python Dictionary.
- json.dumps() again converts our results into JSON formatted string.
Common Use Cases for JSON in Python
JSON is used in almost all data interchanging and it is rare to find a Python application that does not use JSON. Here are some common use cases where Python and JSON work hand in hand:
a. Interacting with APIs
Most of the web APIs return data in JSON format and to parse them in Python we use json module. Here’s how you might handle a JSON response from an API:
#write json file in python to interact with APIs
import requests
import json
response = requests.get('https://api.example.com/data')
data = response.json() # Automatically decodes JSON response
print(data)
b. Storing Configuration Files
Most Python applications read and write their configurations from JSON files because JSON is easy to edit. You can load the configuration and see it in the program as well as change it and save it back to a JSON file.
#write json file in python to store configuration files
import json
# Load configuration
with open('config.json', 'r') as config_file:
config = json.load(config_file)
# Modify the configuration
config["debug"] = True
# Save the updated configuration
with open('config.json', 'w') as config_file:
json.dump(config, config_file, indent=4)
c. Data Storage
It is also useful to indenture structured data for further use and store them in a JSON file. Json is particularly useful for storing the state of an application or transferring data from one system to another.
Conclusion
In this blog post, we brought you a tutorial on how to read and write JSON file in Python, how to deal with JSON strings, and how to structure JSON towards better readability. JSON is a valuable and highly versatile data format that is commonly used for data exchange between applications and Python provides convenient tools for working with JSON data by means of the JSON module.
When developing further Python applications you will meet JSON repeatedly- in the case of interacting with API, with configuration files, or when storing data locally. Learning how to read, write, and manipulate JSON with Python will unlock a lot of opportunities for you as a Python developer.