Java Programming Techniques and APIs
Lambda Expression in Java
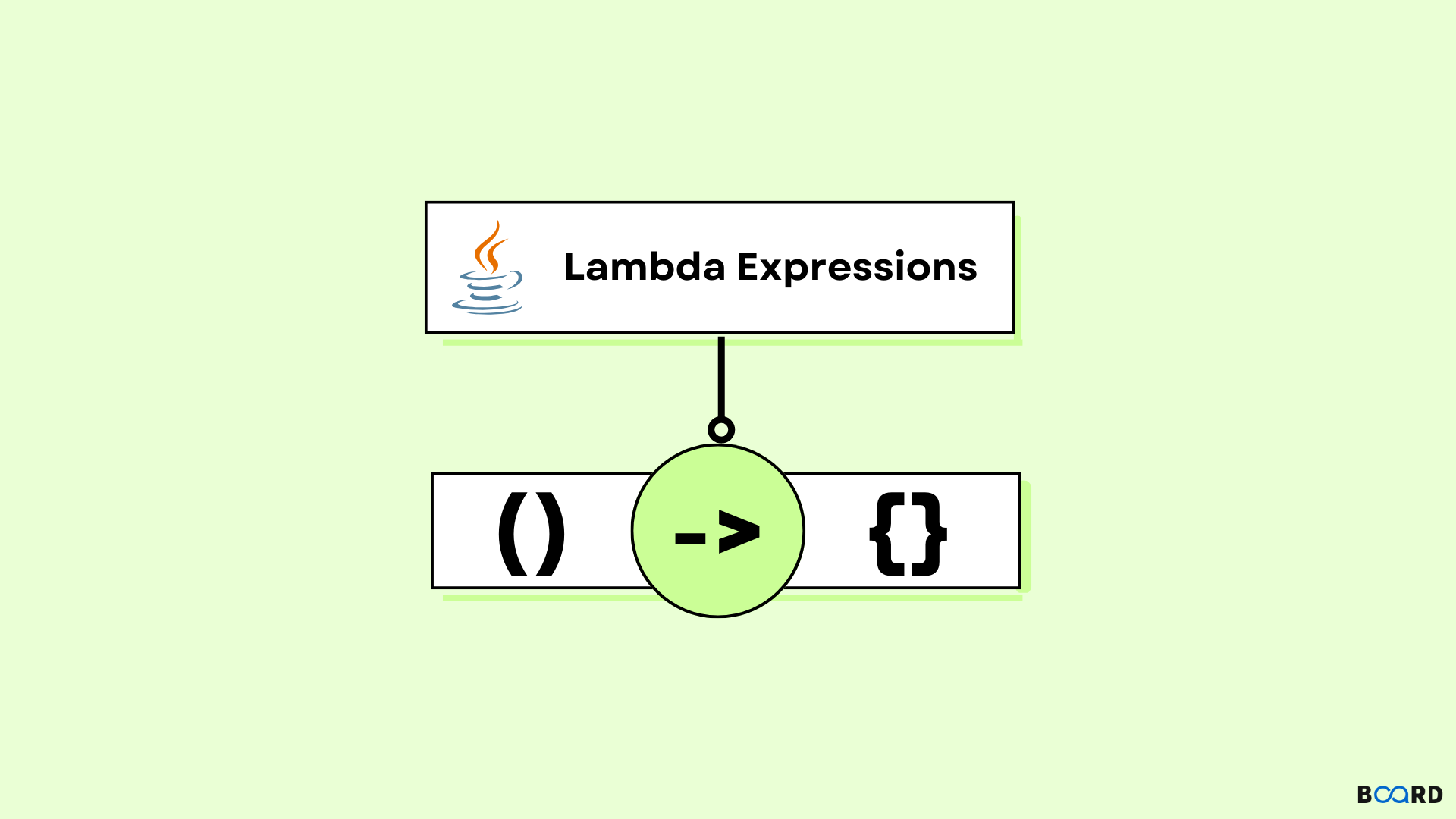
Introduction
In Java SE 8, an important and new feature was introduced Lambda Expressions. Using an expression provides a clear and brief way to express method interfaces. It does help in the collection library as it helps in iterating, filtering and extracting data from a collection.
The compiler doesn’t create a .class file because lambda expression is handled as a function.
Functional Interface
The Functional interface is an interface that only has one abstract method. To declare a functional interface, java provided an annotation i.e. @FunctionalInterface. Lambda expressions give the implementation of a functional interface.
Benefits of Lambda Expression
- Handles the functionality as a method argument or code as data. It saves a lot of code. We do not need to define the method again, for the implementation part, here we just need to type out the implementation code.
- When needed lambda expression can be used as an object and can also be passed around.
- Not being in any class, a function can be created.
- Also gives the implementation of a functional interface.
Java Lambda Expression Syntax
Java lambda expression has three components.
- Argument list: It is empty and also can be non-empty.
- Arrow-token: The arrow token is used to link the body expressions with the arguments list.
- Body: It has the statements for the lambda expressions.
No parameter syntax
One Parameter Syntax
Two Parameter Syntax
No Parameter Java Lambda Expression
Code
Output
Single Parameter Java Lambda Expression
Code
Output
Multiple Parameters Java Lambda Expression
Code
Output
For each loop using Java Lambda Expression
Code
Output
Multiple Statements Java Lambda Expression
Code
Output