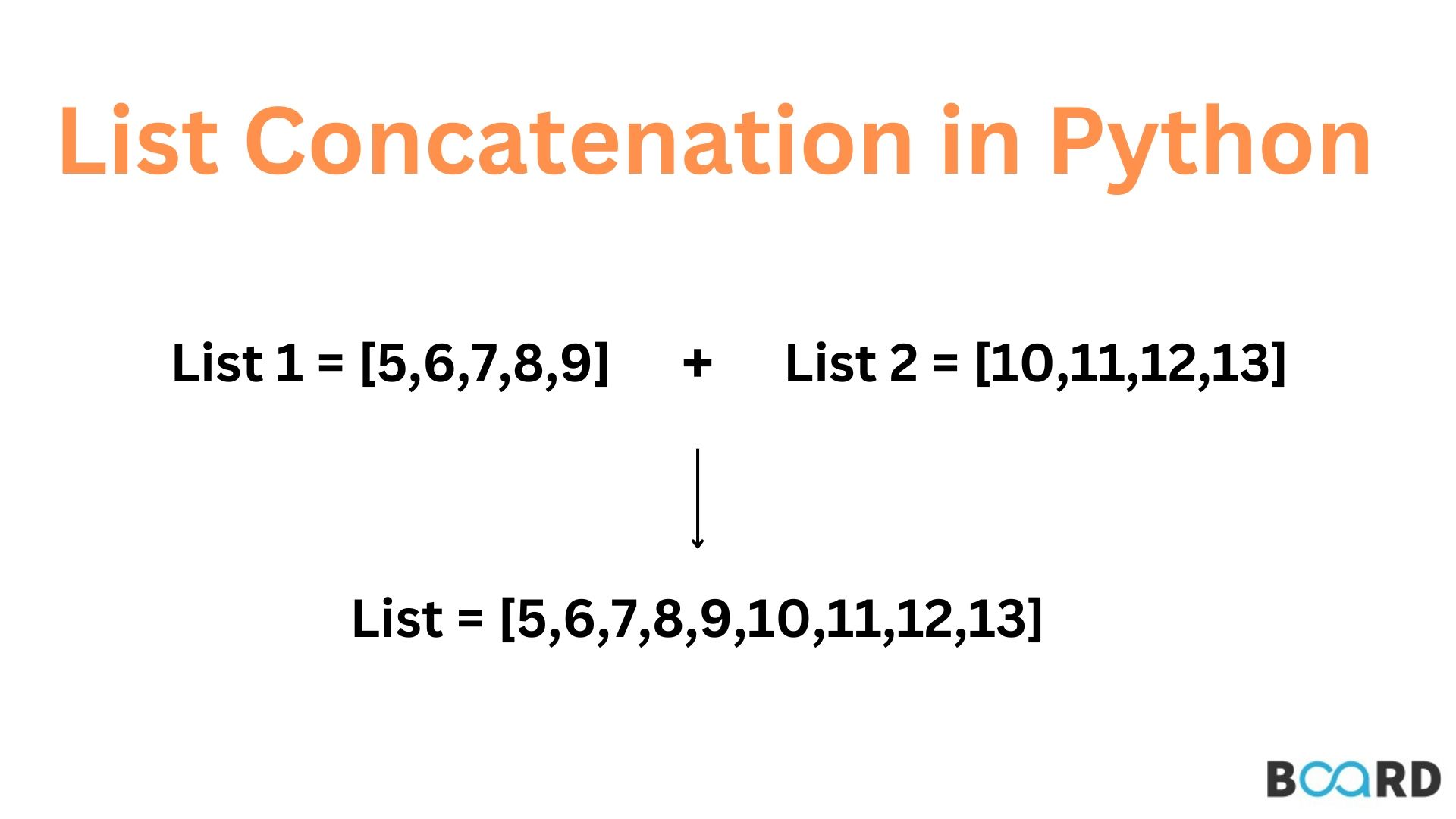
Introduction
This article will show you how to concatenate lists in Python using several techniques. Python Lists are used to store homogenous elements and execute transformations on them. Concatenation, in general, is the process of combining the components of a particular data structure end-to-end. The following are six Python methods for concatenating lists:
List concatenation operator (+)
To concatenate two lists, use the '+' operator. It appends one list to the end of another, resulting in a new list as output.
OUTPUT
Simple List Concatenation Method
A for loop is used in the Naive technique to explore the second list. The entries from the second list are then added to the first list. The first list is the result of concatenating the first and second lists.
OUTPUT
List comprehension to join lists
Python List comprehension is an alternate way in Python for concatenating two lists. List comprehension is creating a list of elements based on an existing list.
It processes the list using a for loop and explores it element by element. The inline for loop below is comparable to a nested for loop.
OUTPUT
List Concatenation with Python's extend() function
To concatenate two lists in Python, use the extend() function. The extend() method iterates through the provided parameter and inserts the item into the list, thus linearly growing the list.
SYNTAX
EXAMPLE
OUTPUT
List Concatenation using Python's '*' operator
The '*' operator in Python may be used to easily concatenate two lists.
In Python, the '*' operator simply unpacks the collection of objects at the index arguments.
Consider the following list: my list = [1, 2, 3, 4].
The statement *my list would replace the list at the index places with its elements. As a result, it unpacks the list of elements.
Example
OUTPUT
To concatenate lists, use Python's itertools.chain() function.
The itertools.chain() function in the Python itertools modules may also be used to concatenate lists.
The itertools.chain() method takes as parameters various iterables such as lists, strings, tuples, and so on and returns a series of them as output.
EXAMPLE
OUTPUT
Thus, in this post, we learned about and developed many methods for concatenating lists in Python.