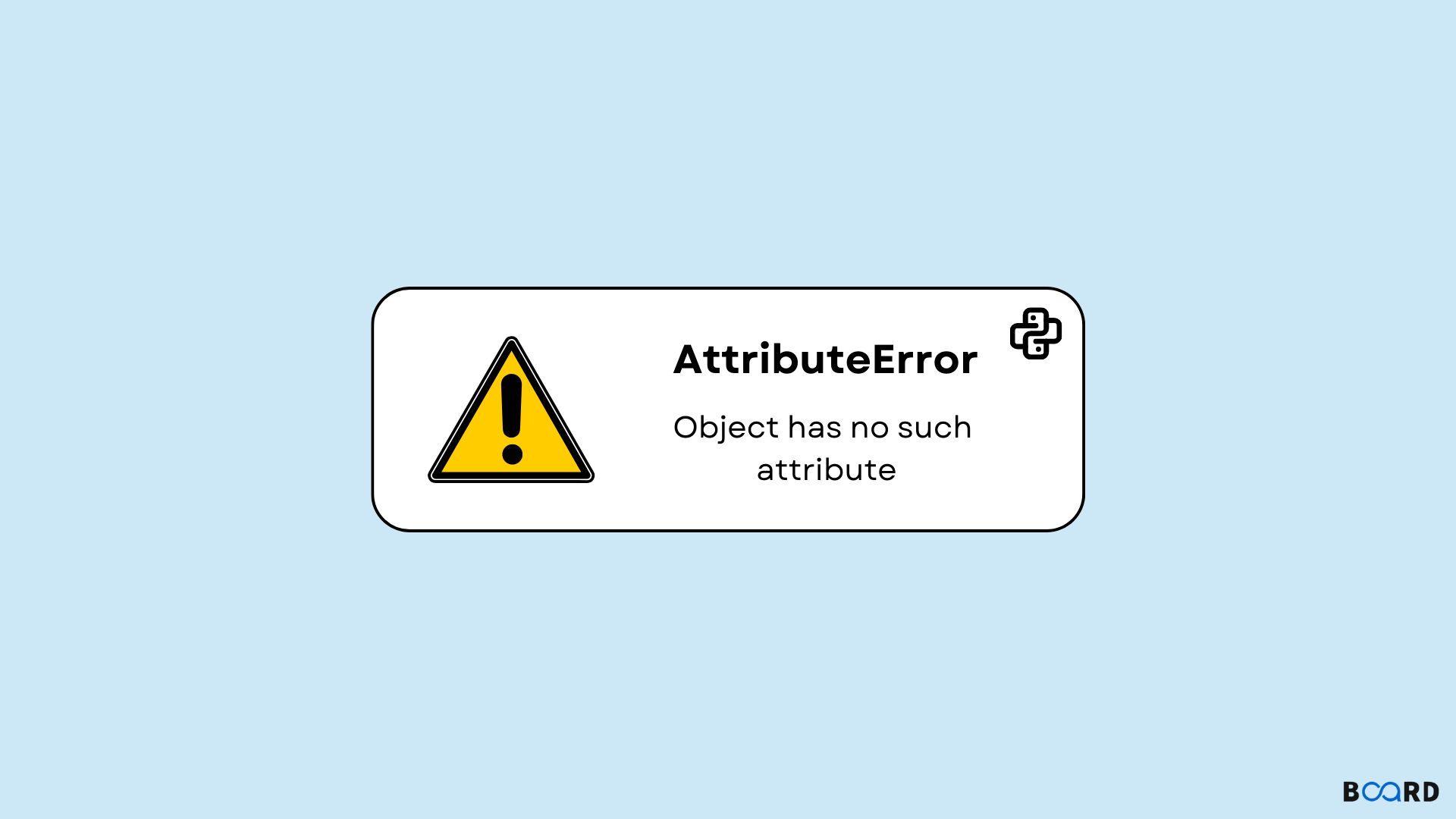
Introduction
A vital component of a programmer's existence are errors. Additionally, receiving an error is not at all negative. Making mistakes indicates that you are learning. But we must fix those mistakes. Furthermore, we need to understand the cause of the mistake before we can fix it. In Python, problems like Type Error, Syntax Error, Key Error, Attribute Error, Name Error, and others are frequently encountered.
What is a Python Attribute Error, why do we receive it, and how do we fix it are all covered in this article. When we attempt to call or access an object's attribute but that object does not have that attribute, the Python interpreter raises an Attribute Error.
For instance, we will encounter an attribute error if we attempt to use higher() on an integer.
Why do we encounter Attribute Error?
An attribute error appears if we attempt to access an attribute that is not owned by that object. For instance, we know that using the capital character will make the string uppercase ().
Output:
Since integers do not have the ability to be upper or lower case, our attempt to convert a number to an upper case letter is unsuccessful. However, we would have received a response if we tried using this upper() on a string since a string can be classified as upper or lower.
Some Common Errors in Python That Cause Attribute Errors
1) If we attempt to conduct an append() operation on a data type other than a List:
Occasionally, when attempting to concatenate two strings, we attempt to append one string into the other, which is not feasible and results in an Attribute Error.
Output:
2) Attempting to access a class attribute that it does not have:
Sometimes, we try to access a class attribute that it does not have. Let's use an illustration to better comprehend it.
In this instance, there are two classes: the Person class and the Vehicle class. Both have unique characteristics.
Output:
3) "NoneType" attribute error
When we receive "None" rather than the instance we anticipate receiving, we see a NoneType Error. It denotes that a task was unsuccessful or produced an unexpected outcome.
Output:
4) When using modules:
When using modules, attribute errors are frequently encountered. Consider that we are importing the hello module and attempting to call two of its functions. Print name() and print age are two examples ().
Module Good day!
Output:
How to Resolve Attribute Error in Python
Use help()
Python's creators have made an effort to address any issue that might arise for Python programmers. If we are uncertain if an attribute belongs to an object in this situation or not, we can employ assistance (). For instance, print(help(str)) will provide a list of all the operations that can be done on strings if we are unsure whether we can use append() on a string. We can use help() on user-defined data types like Class in addition to these built-in data types.
Output:
Isn't it wonderful, for instance, if we have no idea what characteristics the class Person that we defined above has? These are the exact characteristics that we specified in our Person class.
Let's try using help() on our hello module that is included within the hi module now.
Applying the Try-Except Statement
Utilizing try-except statements is a very professional method to handle not only Attribute error but any problem. We can surround a section of code in a try block if we anticipate encountering an error there. Let's try to figure this out.
If we don't know for sure whether the Person class contains the engine type field, we can put it inside a try block.
Output:
Conclusion
In Python, we get an Attribute Error if we attempt to access an attribute that does not belong to the object. Either the help() function or try-except statements can be used to solve the problem.