Vector Erase() and Clear in C++: A Comprehensive Guide
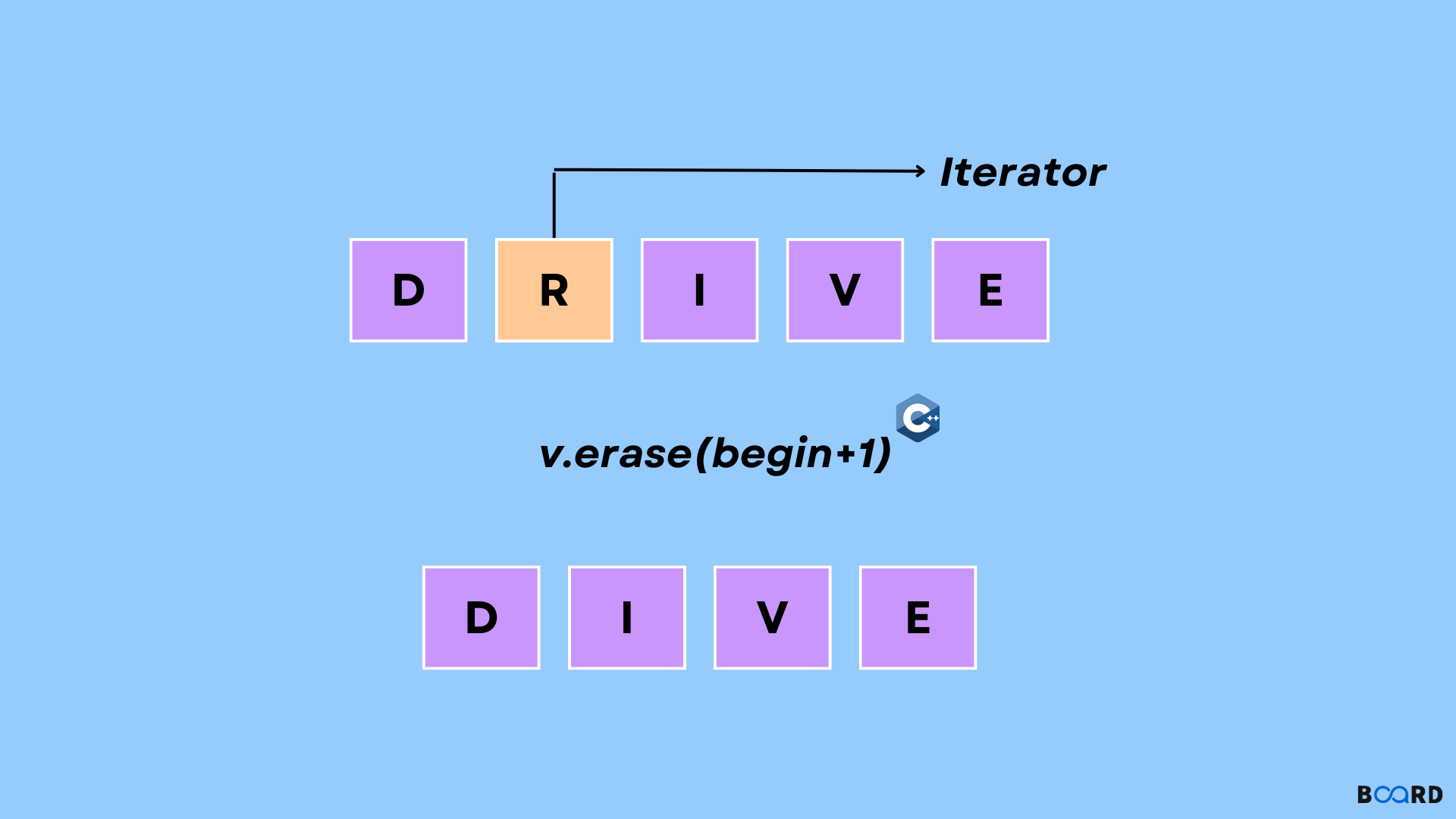
In C++, the std::vector class is one of the elementary structures of data type for storing sequences of elements. It is a container class in the C++ Standard Library and appears in ANSI C++ Libraries. It has a dynamic size, which enlarges or decreases in size depending on the addition or removal of various elements. Unfortunately, efficient memory management and different tricks regarding the manipulation of elements in the vector are required to produce optimized code.
In this blog post, we will dive deep into two important member functions of the std: erase() and clear(). These functions assist in navigating the items of a vector hence facilitating for erasing or even clearing the elements.
Finally, there is the comparison between the erase() and the clear() functions. Moreover, there is a discussion of some issues depending on implementation and specific application of the solution, the basic use cases, and conditions for its fast functioning irrespective of other applications on the device. If you read this blog till the end, you will understand when and how to use erase() and clear() without getting confused.
What is a C++ Vector?
Before delving into erase() and clear(), let’s first briefly review what a std::vector is.
A std::vector is a dynamic array whose size can be increased or decreased with respect to the number of elements that the user wishes to store in it. Vectors also allocate and deallocate memory to themselves, whereas the other data structures let you do this yourself, and elements in vectors are random.
Vectors are widely used because of their ability to extend and shrink and the number of operations required to add and delete elements.
Example
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
Output:
1 2 3 4 5
Using std::vector::erase() to remove an element from a vector in C++
In a vector, the erase() function is used to delete one or more elements from the vector container. However, unlike the clear() function, this one requires knowledge of the iterator because it provides greater flexibility in decision-making on what to erase.
Syntax of erase()
iterator erase(iterator position);
iterator erase(iterator first, iterator last);
- iterator position: The iterator points to the element to be erased.
- iterator first, iterator last: A range of elements to be erased. The function removes elements from vectors in C++ starting from the first and ending at the last (exclusive).
Single Element Erasure
You can use erase() to remove an element from a vector in C++ for any one element at some specific position in the vector. This is well done by passing an iterator that points to the element that is to be erased.
Example
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
// Remove the element at position 2 (third element)
vec.erase(vec.begin() + 2);
// Print the modified vector
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
Output
1 2 4 5
Here the element at index 2 (that is 3) is deleted from the vector.
Range of Elements to Erase
You can also remove a range of elements from a vector using C++ by providing two iterators: one pointing to the first element which is going to be deleted, and the second pointing to one position right of the last element which is going to be deleted.
Example
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
// Remove elements from vector using c++ on position 1 to position 3 (exclusive)
vec.erase(vec.begin() + 1, vec.begin() + 3);
// Print the modified vector
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
Output
1 4 5
Here, elements at index 1 and 2 are deleted meaning elements 2 and 3 are deleted.
Using std::vector::clear() in C++
To remove a number of elements, a method erase() is preferable to clear(), which empties the vector deleting all of its elements.
Syntax of clear()
void clear();
The clear() function unlike the other functions as seen does not have any parameter that is used when it is called. Still, it clears out all items from the vector, and so the vector’s size becomes zero.
Example of clear()
#include <iostream>
#include <vector>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
// Clear all elements from the vector
vec.clear();
// Print the vector size
std::cout << "Size after clear: " << vec.size() << std::endl;
return 0;
}
Output
Size after clear: 0
In this case, all elements are erased and the vector is empty with size zero.
Differences Between erase() and clear()
Despite erasing meaning the same as clearing in literal terms and both being functions to remove one or several elements from a vector, they are used in different situations.
Feature | erase() | clear() |
Purpose | Deletes one or several items at particular index or indices | Erase all values inside the vector |
Parameter | Usually takes one elements as arguments, as well as a range of elements | Takes no parameters |
Efficiency | Delete given values in list and advances the rest elements | It deletes all elements in the list with out shifting. |
Vector Size After Call | Reductions by the amount of removed elements | Becomes 0 |
In short, we use erase() to remove elements from vectors in C++ when you have to remove a certain element or set of elements and employ the function clear() when we wish to erase all the elements in the vector.
Performance Considerations
While using vectors, let’s remember that nuking (deleting) items has some performance consequences to discuss.
Erase Operation
The erase() function is said to have a worst-case time complexity of O(n) where n is the number of elements next to the erased element. This is because all elements after the erased form of a linked list have to be shifted in order to fill the space created by the removal. Erase() should not be used within a loop when dealing with large vectors as it causes bottlenecks.
Clear Operation
The clear() function, without any exception, takes O(n) time where n is the number of elements in a vector. However, while with erase() some elements must be shifted as this function removes only a few elements whereas clear() erases all elements of the vector and sets the size to 0. This can be more efficient when for example, one wants to just have the vector as a receptacle to put something and take it out later.
Best Practices
Avoid Erasing in Loops:
In case, you employed the process of eradicating elements within a loop you should consider the cost to shift elements after each deletion. Erase returns an iterator pointing to the new end of the vector, preferably iterate vectors with iterators that aren’t sensitive to the vector resizing. Example of safe removal in a loop:
for (auto it = vec.begin(); it != vec.end();) {
if (*it == value_to_remove) {
it = vec.erase(it); // Returns iterator to the next element
} else {
++it;
}
}
Reserve Space
If you know that the vector is going to extend greatly, try to do the memory allocation with the reserve() function in advance. Although this does not have any effect on erasing and clearing it helps to avoid unnecessary reallocation when using the insert member.
Use clear() When Appropriate
In case when you need to remove all elements, you should use the clear method instead of the erase() method as this one works faster.
Conclusion
In this blog post, we’ve explored two essential member functions of std::vector: erase() and clear(). Both of these functions are quite important in managing and manipulating the contents of the vector, and the identification of the right function is important as their implementation would consequently result in the generation of more efficient and easier code.
- erase() is suitable for freeing the specified elements or ranges of elements in a vector.
- If you need to clear the vector and delete all of its elements use clear().
- Performance must be taken into account when removing blocks of elements, delete inside loops should be avoided in optimal time.
By learning these functions, you will be able to write C++ vector erase code more efficiently while writing cleaner code than other programmers.